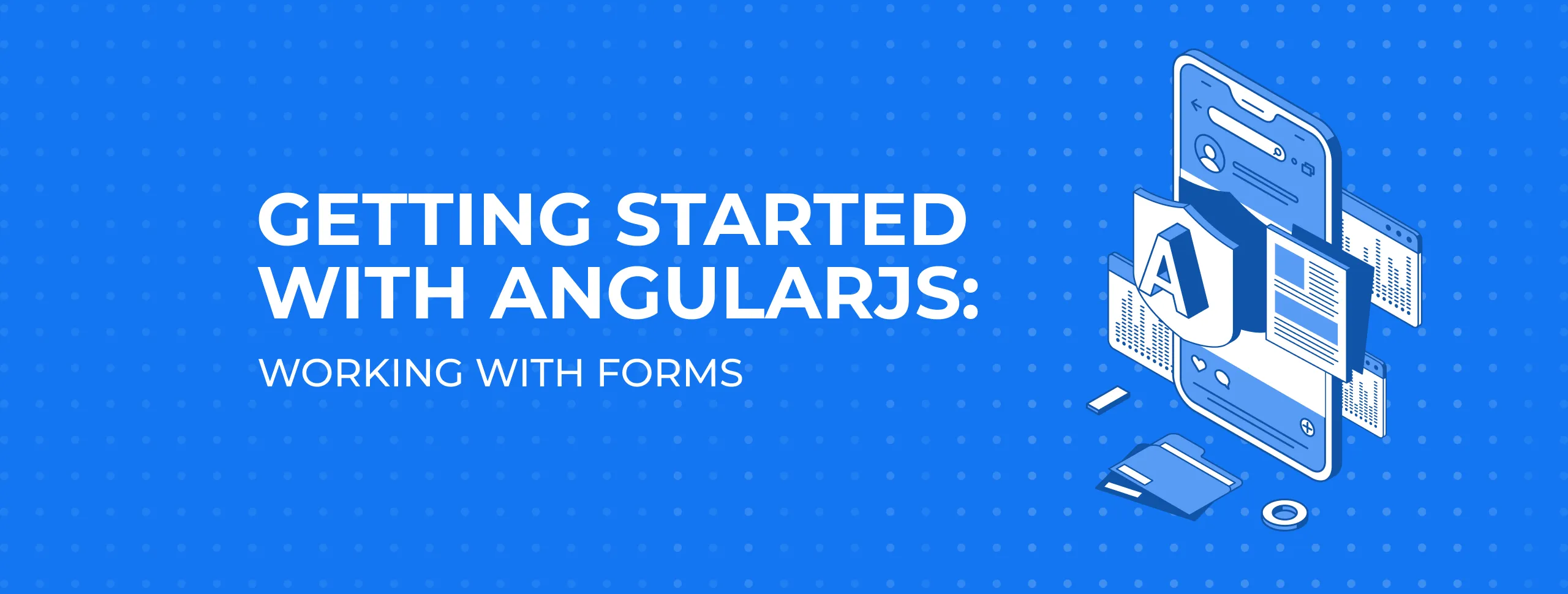
Getting Started with AngularJS: Working with Forms
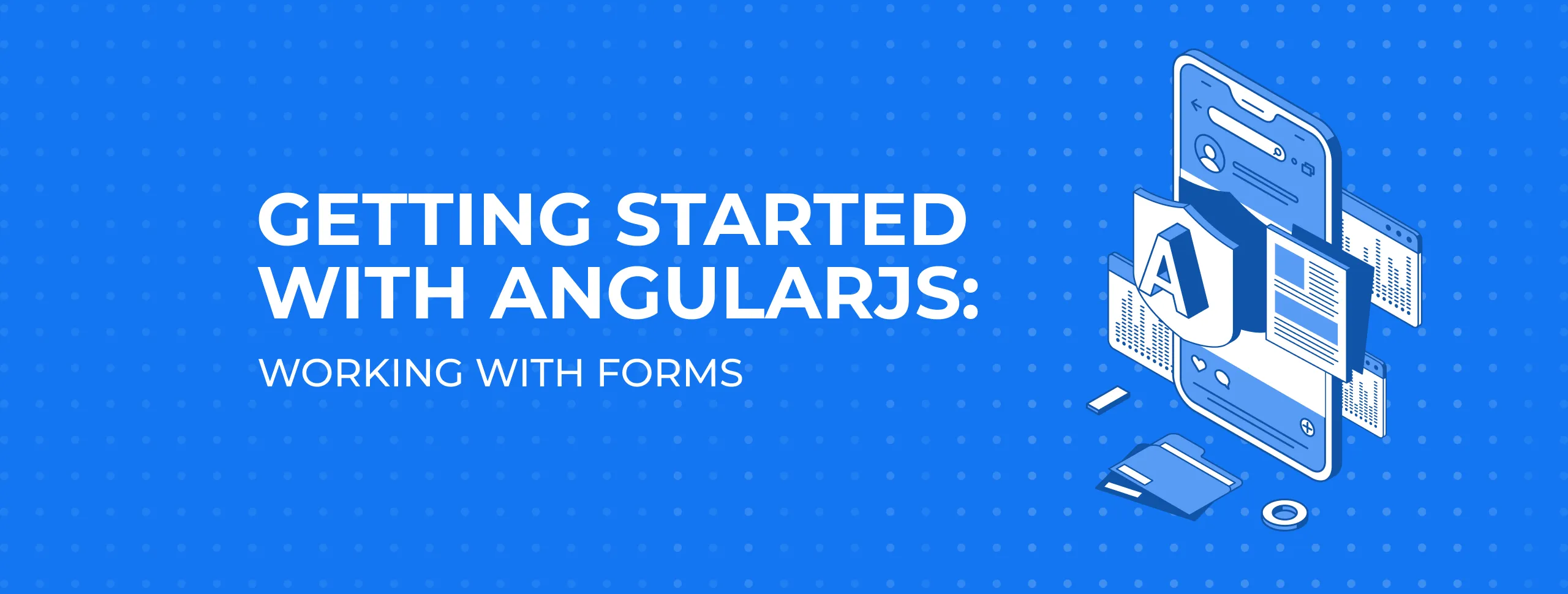
Hello again! As promised, here's a post to follow my previous article. This time, I'll talk about a way of eliminating the necessity to write boilerplate code for your projects.
The basics
AngularJS is a framework that supports module structure, which allows creating independent modules. Let’s break down the approach using forms as an example. Now, what is required for forms to work?
- View - for displaying fields
- Validation - for checking input data
- Data processing
The first two items will appear in every project, as 90% of forms look alike. Let’s make a form into a component that will take care of display and validation. Component responsible for validation we’ll get from our repository. We can divide form generator into 2 modules:
- The first one is the form itself. It will process our data object and launch function, if the form is filled out correctly.
- The second one is responsible for field rendering and validation.
this.model = { url: 'sign-in', submit: function (data) { }, rules: { email: { type: 'invalid', message: 'Email error', rule: function (form, field) { var re = /^([\w-]+(?:\.[\w-]+)*)@((?:[\w-]+\.)*\w[\w-]{0,66})\.([a-z]{2,6}(?:\.[a-z]{2})?)$/i; return field.$dirty && !re.test(field.$modelValue); } } }, attr: { identifier: { type: 'text', attr: {placeholder: 'Your email or phone number'}, validators: ['required', 'email'] }, gender: { type: 'select', value: 'man', options: [{ name: 'Man', value: 'man' }, { name: 'Woman', value: 'woman' }], attr: {placeholder: 'Your email or phone number'}, validators: ['required'] }, password: { type: 'password', attr: {placeholder: 'Your password'}, validators: ['required'] } }, buttons: { submit: { text: "Sign in" } } };
body div(ng-controller="demoCtrl as ctrl") mgr-form-builder(model="ctrl.model")
scope: { model: '=', formName: '=', templateUrl: '@' }, templateUrl: function (el, attr) { if (attr.templateUrl) { return attr.templateUrl; } return 'formBuilder/formBuilder.html'; },
formBuilderCtrl.submit = function () { if (!formBuilderCtrl.model.submit) { return false; } var data = {}; Object .keys(formBuilderCtrl.model.attr) .forEach(function (key) { data[key] = formBuilderCtrl.model.attr[key].value; }); formBuilderCtrl.model.submit(data); };
Result
We have a component that we can use from project to project and we don't have to worry about how or what to validate. We just need to describe data model. Want to learn more? Take a peek into our repository.related
recent
recommended